Bob's Journey into Secrets and Config Maps
Join Bob as he navigates Kubernetes Secrets and ConfigMaps under Josie’s guidance. Learn how to securely manage sensitive data and configurations while separating them from application code, ensuring a robust and secure Kubernetes setup. Another step forward in Bob’s journey!

Fresh off his success securing his Kubernetes pods, Bob turned his attention to a critical aspect of application security and configuration: secrets and ConfigMaps management. He recalled the Kubernetes secrets he'd created with Alex and realised he needed a deeper understanding of how to protect these sensitive pieces of information, as well as how to manage configurations effectively.
"Secrets are like the keys to the kingdom," he mused, "but how do I ensure they're truly protected? And how can I manage my application's configurations without hardcoding them?"
As if on cue, Josie appeared, a knowing smile on her face. "Thinking about secrets and configurations, Bob?" she asked.
Bob nodded. "I know they're important, but I'm not sure I fully grasp the best practices for managing them."
Josie pulled up a chair, her expression thoughtful. "Before we dive in, Bob, let me ask you ... what would you do to keep secrets like API keys safe in Kubernetes?"
Bob paused, considering. "I’d probably start with encryption, but beyond that, I’m not sure." Josie nodded approvingly. "Encryption is a great start, but there’s more to it. Let’s explore together."
📂 Code Repository: Explore the complete code and configurations for this article on GitHub.
Kubernetes Secrets
"So, what exactly are Kubernetes secrets?" Bob asked, eager to clarify his understanding.
Josie explained that Kubernetes Secrets provided a secure way to store and manage sensitive information, such as passwords, API keys, and certificates.
"They're like the vaults of your Kubernetes cluster," she explained, "protecting your valuable assets."
Bob learned that secrets are stored in etcd, the key-value store that acts as the brain of a Kubernetes cluster. It's where Kubernetes keeps track of all its critical information, from pod deployments and configurations to service definitions and, yes, even secrets.
Josie explained, "Etcd is like a highly secure and reliable library where Kubernetes stores all its important records. Because secrets are stored in etcd, protecting access to etcd is paramount. It's like keeping the library under lock and key, with only authorised librarians allowed in."
She emphasised the importance of securing etcd with:
- Proper authentication: Ensuring that only authorised components and users can access etcd. This is like having a strict ID check at the library entrance
- Authorisation: Implementing fine-grained access controls to restrict what each user or component can access within etcd. This is like giving librarians different levels of access to sections of the library
- Encryption: Encrypting the data stored in etcd to protect it from unauthorised access, even if the underlying storage is compromised. This is like keeping the most valuable books in the library in secure, encrypted vaults
"By securing etcd," Josie concluded, "you're not just protecting secrets but also ensuring the integrity and availability of your entire Kubernetes cluster. It's the foundation of a secure and reliable Kubernetes environment."
Best Practices for Secret Management
"What are some of the best practices for managing secrets in Kubernetes?" Bob inquired, keen to learn how to safeguard his application's sensitive data.
Josie then guided Bob through some best practices for managing secrets in Kubernetes:
- Use Strong Values: Generate random, hard-to-guess secrets i.e. avoid defaults
- Apply Access Controls: Restrict access using RBAC so only authorised users and pods can retrieve secrets
- Rotate Regularly: Update sensitive secrets periodically to reduce exposure risks
- Avoid Hardcoding: Use environment variables or volumes to pass secrets to applications, never directly in code
- Leverage External Tools: For advanced use cases, integrate tools like HashiCorp Vault or AWS Secrets Manager for encryption and auditing
Secret Types
"Are all secrets the same?" Bob asked, curious about the different ways secrets could be used.
Josie explained that Kubernetes supports different types of secrets:
- Opaque: The default type, suitable for storing general secrets as key-value pairs
- kubernetes.io/dockerconfigjson: Used to store Docker registry credentials for pulling images
- tls: Used to store TLS certificates and keys for secure communication
Secrets in Action
Josie then showed Bob how to create a secret using a YAML definition:
apiVersion: v1
kind: Secret
metadata:
name: my-database-password
type: Opaque
data:
password: <BASE64_ENCODED_PASSWORD>
She explained how to mount the secret as an environment variable in a pod:
apiVersion: v1
kind: Pod
metadata:
name: my-pod
spec:
containers:
- name: my-container
image: my-image
env:
- name: DATABASE_PASSWORD
valueFrom:
secretKeyRef:
name: my-database-password
key: password
"Now, your application running in the pod can access the database password securely," Josie concluded. "That's handy," Bob remarked, "but what about other types of secrets?" "Good question," Josie replied. "Let's look at how to store Docker registry credentials for pulling private images."
apiVersion: v1
kind: Secret
metadata:
name: my-docker-registry-secret
type: kubernetes.io/dockerconfigjson
data:
.dockerconfigjson: <BASE64_ENCODED_DOCKERCONFIGJSON>
"The .dockerconfigjson
field contains a Base64-encoded config.json
file, which holds your registry credentials," Josie explained. Bob nodded, following along. "And how about TLS certificates for secure communication?" Josie smiled. "Kubernetes has a specific type for that too."
apiVersion: v1
kind: Secret
metadata:
name: my-tls-secret
type: kubernetes.io/tls
data:
tls.crt: <BASE64_ENCODED_CERTIFICATE>
tls.key: <BASE64_ENCODED_PRIVATE_KEY>
"This secret type allows you to store your TLS certificate and private key securely," Josie explained. "You can then use it to enable HTTPS for your services."
Bob was impressed by the flexibility and security that Kubernetes Secrets offered. He felt confident that he could now manage and protect his application's sensitive data effectively.
Bob, having explored the intricacies of Kubernetes secrets, felt a sense of accomplishment. He now understood how to securely store and manage sensitive information in his Kubernetes deployments.
"Secrets management is like a hidden layer of protection," he mused, "ensuring that my application's crown jewels remain safe."
Josie nodded in agreement. "It's a critical aspect of Kubernetes security that often gets overlooked. By following best practices and utilising the various secret types, you can significantly enhance the security posture of your applications."
- Kubernetes Secrets provide a secure way to store and manage sensitive information in your cluster
- Protecting etcd, the key-value store where secrets are stored, is crucial for overall cluster security
- Employing strong secret creation practices, access control, and regular rotation are essential for minimising risks
- Avoid hardcoding secrets in your application code or configuration files
- Consider using external secrets management solutions for advanced security features and centralised control
- Kubernetes offers various secret types to cater to different needs, such as storing database passwords, Docker registry credentials, and TLS certificates
Bob knocks up a quick diagram to reinforce his learning and asks "Is this how the relationships work?"
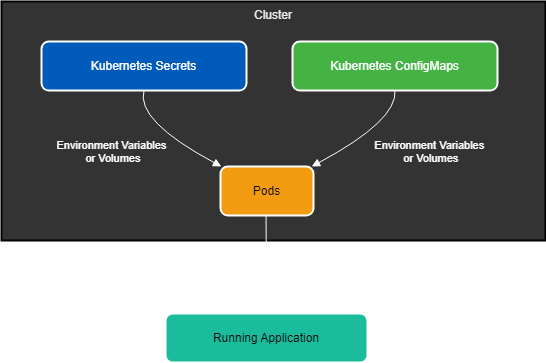
Josie nod's in approval, "That's pretty good, but remember secrets can also be used for certificates and dockerconfigs so may not be directly mounted into a pod".
Bob, armed with this knowledge, was ready to tackle the next challenge in his Kubernetes journey, confident that his applications' secrets were well-protected.
Kubernetes ConfigMaps
Josie then introduced Bob to another essential Kubernetes concept: ConfigMaps.
"ConfigMaps are like dictionaries for your applications," she explained. "They store configuration data separately from your application's code, making it easier to manage and update."
Bob learned that ConfigMaps can hold various types of configuration data, such as environment variables, command-line arguments, and configuration files. He also discovered that ConfigMaps can be mounted as volumes or injected as environment variables into pods, providing flexibility in how applications access configuration data.
Josie explained why ConfigMaps are so valuable in Kubernetes deployments.
Benefits of Using ConfigMaps
Josie highlighted the benefits of using ConfigMaps:
- Separation of Concerns: ConfigMaps separate configuration from code, making it easier to manage and update configurations without modifying the application's code
- Flexibility: ConfigMaps can be used to store various types of configuration data and can be accessed by applications in different ways
- Version Control: ConfigMaps can be version-controlled, allowing for easy rollback to previous configurations if needed
- Portability: ConfigMaps make it easier to move applications between different environments, as the configuration data is stored separately and can be easily updated
ConfigMaps in Action
Josie then showed Bob how to create a ConfigMap using a YAML definition:
apiVersion: v1
kind: ConfigMap
metadata:
name: my-config
data:
database_host: my-database.example.com
database_port: "3306"
"We can mount this ConfigMap into our pods by include a new volumeMount as follows" Josie said:
apiVersion: v1
kind: Pod
metadata:
name: my-pod
spec:
containers:
- name: my-container
image: my-image
volumeMounts:
- name: config-volume
Bob, now well-versed in the intricacies of secrets and ConfigMaps, felt a growing sense of confidence in his ability to manage sensitive data and configurations within his Kubernetes deployments. He had learned how to protect his application's secrets, ensuring they remained confidential and secure. He had also discovered the power of ConfigMaps in separating configuration from code, making his deployments more flexible and maintainable.
"Secrets and ConfigMaps are essential tools in the Kubernetes toolbox," he remarked, appreciating their role in building secure and well-organised applications. Josie nodded in agreement. "They're often overlooked, but they play a crucial role in ensuring your applications run smoothly and securely."
With Secrets and ConfigMaps securely integrated, Bob knew he was one step closer to a fully operational system. He couldn’t wait to explore how traffic routing mechanisms like Endpoints would contribute to the bigger picture. The journey continued, with Josie leading the way.
But Bob's adventure wasn’t over yet. He knew another critical aspect of Kubernetes deployments awaited: persistent storage.
"What about data that needs to persist even if my Pods are deleted or rescheduled?" Bob wondered aloud.
Josie smiled, sensing his eagerness to learn more. "Persistent storage is a game-changer," she said. "It's how you ensure critical data survives Pod restarts or rescheduling. Let's explore that next."
Stay tuned for Part 7, where Bob dives into the world of Persistent Volumes and discovers how to manage persistent data securely in his Kubernetes deployments.